How to stream your radio station on Alexa
A tool to help you stream your radio station to Amazon’s Alexa devices.
Used by Internet, AM, FM and DAB stations around the world!
Not technical? Don’t worry. Every step is covered.
If you can cut and paste you have this covered.
One to one guidance and help available.
Step 1. – Pick Station Name, Website and stream address
Step 2. Adjust below code to suit the previous choices
Below is the JSON you will use as a baseline and adjust for your own names, etc
{
"interactionModel": {
"languageModel": {
"invocationName": "radio tincup",
"intents": [
{
"name": "AMAZON.CancelIntent",
"samples": [
"Cancel"
]
},
{
"name": "AMAZON.HelpIntent",
"samples": [
"Help"
]
},
{
"name": "AMAZON.StopIntent",
"samples": [
"Stop"
]
},
{
"name": "Play",
"samples": [
"Ask radio tincup to play radio tincup",
"Start radio tincup",
"Ask radio tincup to play",
"Play radio tincup"
]
},
{
"name": "AMAZON.NavigateHomeIntent",
"samples": []
},
{
"name": "AMAZON.PauseIntent",
"samples": []
},
{
"name": "AMAZON.ResumeIntent",
"samples": []
}
],
"types": []
}
}
}
Below is the Javascript for your skill to use as a base, you will adjust names, etc in this as well
const Alexa = require('ask-sdk-core');
var https = require('https');
const PlayHandler = {
canHandle(handlerInput)
{
return (
handlerInput.requestEnvelope.request.type === 'LaunchRequest' ||
(
handlerInput.requestEnvelope.request.type === 'IntentRequest' &&
handlerInput.requestEnvelope.request.intent.name === 'Play'
) ||
(
handlerInput.requestEnvelope.request.type === 'IntentRequest' &&
handlerInput.requestEnvelope.request.intent.name === 'AMAZON.ResumeIntent'
)
);
},
handle(handlerInput)
{
return handlerInput.responseBuilder
.addDirective({
type: 'AudioPlayer.Play',
playBehavior: 'REPLACE_ALL',
audioItem:{
stream:{
token: '0',
url: 'https://radio.iandreev.com/myradio.ogg',
offsetInMilliseconds: 0
}
}
})
.getResponse();
}
};
const PauseStopHandler = {
canHandle(handlerInput)
{
return (
handlerInput.requestEnvelope.request.type === 'IntentRequest' &&
(
handlerInput.requestEnvelope.request.intent.name === 'AMAZON.CancelIntent' ||
handlerInput.requestEnvelope.request.intent.name === 'AMAZON.StopIntent'
)
) ||
(
handlerInput.requestEnvelope.request.type === 'IntentRequest' &&
handlerInput.requestEnvelope.request.intent.name === 'AMAZON.PauseIntent'
);
},
handle(handlerInput)
{
return handlerInput.responseBuilder
.addDirective({
type: 'AudioPlayer.ClearQueue',
clearBehavior: 'CLEAR_ALL'
})
.getResponse();
}
};
const HelpIntentHandler = {
canHandle(handlerInput)
{
return handlerInput.requestEnvelope.request.type === 'IntentRequest' &&
handlerInput.requestEnvelope.request.intent.name === 'AMAZON.HelpIntent';
},
handle(handlerInput)
{
const speechText = 'You can say Play, Stop or Resume. For more information please visit radio dot iandreev dot com';
return handlerInput.responseBuilder
.speak(speechText)
.getResponse();
}
};
const SessionEndedRequestHandler = {
canHandle(handlerInput)
{
return handlerInput.requestEnvelope.request.type === 'SessionEndedRequest';
},
handle(handlerInput)
{
return handlerInput.responseBuilder.getResponse();
}
};
const IntentReflectorHandler = {
canHandle(handlerInput)
{
return handlerInput.requestEnvelope.request.type === 'IntentRequest';
},
handle(handlerInput)
{
const intentName = handlerInput.requestEnvelope.request.intent.name;
const speechText = 'NO INTENT HELP TEXT';
return handlerInput.responseBuilder
.speak(speechText)
.getResponse();
}
};
const ErrorHandler = {
canHandle()
{
return true;
},
handle(handlerInput, error)
{
const speechText = `Sorry, I could not understand what you said. Please try again.`;
return handlerInput.responseBuilder
.speak(speechText)
.reprompt(speechText)
.getResponse();
}
};
exports.handler = Alexa.SkillBuilders.custom()
.addRequestHandlers(
PlayHandler,
PauseStopHandler,
HelpIntentHandler,
SessionEndedRequestHandler,
IntentReflectorHandler)
.addErrorHandlers(
ErrorHandler)
.lambda();
Step 3: Create a free Amazon Developer Account
Go to https://developer.amazon.com/ and sign in
Step 3: Create a free Amazon Developer Account
Go to https://developer.amazon.com/ and sign in or create an account.
Step 4: Create an Alexa Skill
Go to https://developer.amazon.com/alexa/console/ask and click the Create Skill button.

Then enter Your Station Name in the Skill Name form field, and select the Default Language of your skill.

Next you need to Choose a model to add to your skill, select the Custom option.
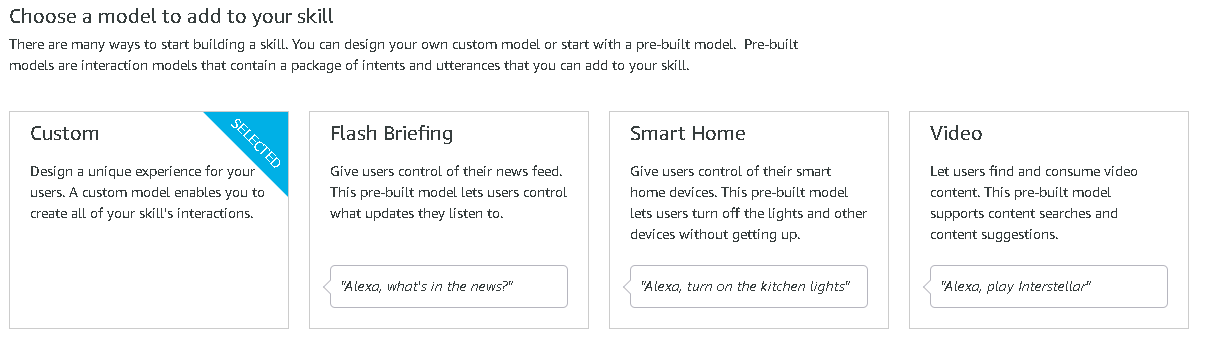
Now Choose a method to host your skill’s backend resources, and select the Alexa-Hosted (Node.js) option.
The Alexa-Hosted (Node.js) option comes with a rather generous helping of free computational power and storage space, which should be more than enough for most online radio stations:
“The following limits apply to each account. AWS Lambda: 1 million free AWS Lambda requests and 3.2 million seconds of compute time per month. Amazon S3: 5 GB of Amazon S3 storage, 20,000 get requests, 2,000 put requests, and 15 GB of data transfer out per month.” – Usage Limits

Scroll towards the top of that page, click the Create Skill button up there.
January 2020 Update: An extra step!
The new step of Choose a template to add to your skill, has caused confusion for several people who emailed me recently; just select the Hello World Skill option and click Choose: don’t worry about us not actually wanting a Hello World Skill, we’re going to overwrite everything it does next.
It may take Amazon a couple of minutes to create your skill, I’d suggest grabbing a cuppa whilst you wait.

Once this is done you’ll get several alerts on the right hand side of your scren, don’t worry about these, click the Xs to clear them and you should be good to continue.
Step 5: Enable the AudioPlayer Interface
Because playing a stream requires what Amazon calls the AudioPlayer Interface, we’ll need to enable it.
On the left hand side of the screen, there should be a menu, click Interfaces, then enable the Audio Player.

Now click the Save Interfaces, button near the top of the screen.

Step 6: Paste the JSON
Now click the JSON Editor link from the menu on the left.
Select all of the code in the textarea, then delete it.

Copy the JSON code from this page, paste it into the textarea.
Click the Save Model button at the top of the screen, followed by the Build Model button.

Step 7: Paste the JavaScript
Next click the Code link from the menu at the top of the screen. This will open up index.js in the JavaScript code editor.
Like before, select the JavaScript code in the form; delete it, then paste the new JavaScript from this page in its place.
Next click the Save, button, followed by the Deploy button.
Step 8: Enable Testing
Before you can test this on your Alexa device, you need to click the Testing link from the menu at the top of the screen.
Switch on Testing.

Step 9: Device Testing
Whilst Amazon provide an excellent browser based tool for testing your skill, it doesn’t play any audio so you’re better off testing it with a real gadget; that is after all what you’re listeners will be tuning in on!
If your Alexa device is registered to the same account as your Amazon Developer account, you will now have access to your radio station on your Alexa.
Say “Alexa, play Your Station Name”
You should now be listening to your streaming radio station!
Time for a little celebration
I’ve released this tutorial for free, it’s taken a lot of hours to figure our how Amazon’s systems work, and even longer to write up a tutorial and code generator, it’s also taken more time to keep up with Amazon’s changes.
If you’re listening to your radio station on your Alexa device now, please share some joy!
Step 10: Distribution
Consider these final few steps the paperwork you need to complete to get your new skill listed, and available for the public to tune into.
Skill Preview
This defines how your skill will appear to customers on the Amazon site.
Amazon need you to complete the following for your skill’s public page.
- Public Name
- One Sentence Description
- Detailed Description
- What’s New
- Example Phrases
- Small and Large Skill Icons
- Category
- Keywords
- Privacy Policy URL
- Terms of Use URL
Privacy and Compliance
- Does this skill allow users to make purchases or spend real money?
- Does this Alexa skill collect users’ personal information?
- Is this skill directed to or does it target children under the age of 13?
- Does this skill contain advertising?
- Export Compliance
- Testing Instructions
Advertising: Amazon allow advertising, as long as the ads meet the criteria below; this forbids giving Alexa a dedicated stream, and serving different ad output than you do on your website, or app: some might find this a little restricting, as a former Group Traffic Manager, I used to send different ad breaks to different transmitters, and it makes sense for a station to utilise different media for different campaigns, but that’s more advanced than your average streaming setup!
“Streaming music, streaming radio, podcast, and flash briefing skills may include audio advertisements as long as (1) the advertisements do not use Alexa’s voice, Amazon Polly voices, or a similar voice, refer to Alexa, or imitate Alexa interactions and (2) the skill does not include more or materially different advertising than is included when the same or similar content is made available outside of Alexa.”
Testing Instructions:
In order to pass Amazon Certification you need to test the following voice commands, then once satisfied your stream behaves as it should, paste these details into the form:
- Alexa, Play Your Radio Station
- Alexa, Pause
- Alexa, Resume
- Alexa, Start Your Radio Station
- Alexa, Stop
- Alexa, Ask Your Radio Station to play
- Alexa, Cancel
- Alexa, Ask Your Radio Station to play Your Radio Station
Pro-Tip: Paste a link to an online copy of your music licences in the testing field
Whilst Amazon are thorough, and will ask you to provide copies of your music licences, I find it interesting knowing the TuneIn app, and skill, are in no way as conscientious as Amazon with regards to royalties: there are over 100,000 stations on TuneIn, where is the same concern for rights with each of them?
“If your skill makes legitimate use of trademarks, intellectual property, or brands, you must clearly indicate that you have permission to use these, and provide proof, when you submit your skill for certification. In the developer console, go to Distribution > Privacy & Compliance and use the Testing Instructions form to provide this information, including external links to documentation evidencing your right to use the intellectual property (such as proof of trademark registration or a license agreement with the rights owner) and contact information, if needed. With this information, the certification team will be able to determine if your skill has the necessary permissions.”
Source: https://developer.amazon.com/docs/custom-skills/policy-testing-for-an-alexa-skill.html
Availability
This is where you can set who has access to this skill, and which countries it’ll be available in.
This is where you can geo-lock your stream on Alexa, to meet your music licencing requirements.
Step 11: Certification and Submission
You need to click the Certification link from the menu at the top of your screen.
These final certification steps, each launched via the menu on the left, ensure that quality criterea are met before your skill can be launched to the public, it automates a series of tests like checking for complete information; broken code and bad syntax:
Validation
If you skipped any of the questions in the Distribution section the validation report will pick them up and tell you what needs correcting. If only every online tool was as thoroughly validated, and as well explained as this:
This is the screen you want to be looking at now:
Functional Test
So close now, hit that Run button!
![]()
The final step: Submission!
If you’ve followed every step of this guide you should now be ready to submit your Alexa Skill.
Go on, do it: hit that blue button that you’ve been working towards!
Good luck!
Step 12: If this helped, why not buy me a coffee, or give this page a share?
I’ve seen websites offering hosted Alexa Skills for Radio Stations; some of them are £10 a month, if this guide has helped you get your radio station’s stream on Amazon gadgets, and potentially saved your station £120 a year, please consider passing some of your savings my way!
Amazon eGift Card: send to Jlowens76 @ gmail.com
Frequently Asked Questions
Well, not that frequent, but people have been asking:
Can this manage streams for more than one station?
Yes, but you’d need to hack around with the code.
You might just find it a lot easier to create a skill for each station you manage; this will involve having to put each different skill through Amazon’s certification, it requires a little more admin, but it does negate the need for more complex code.
Is there a quick hack to getting Icecast running on HTTPS?
Sorry, no. As for why Amazon insist on SSL streams, I’m too am perplexed about their reasoning.
How do I get Icecast to run on SSL?
CertBot is pretty cool, it creates free SSL Certificates, the Icecast website has a guide to follow, though I may create a blog post about automating the whole process.
What about a guide for streaming via Google Play devices?
Maybe one day… I just don’t want to give Google any cash!
Alexa Help!
I’ve done tutorials like this before and struggled with comment admin; it really can go crazy! Therefore if you’ve any questions or comments please email me, I’ll try to respond to everyone, and will update this page accordingly.
I’d love to know which stations have used it so please feel free to drop me a line and let me know.
Thanks for reading, hope this helps
Original credit: (site down, scraped from internet archive) https://andymoore.info/stream-your-radio-station-on-alexa-for-free (https://web.archive.org/web/20200511134625/https://andymoore.info/stream-your-radio-station-on-alexa-for-free)